JQuery Interview Questions Part - 7
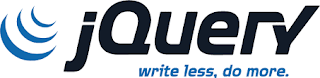
Q 1. What is AJAX?
Ans 1. AJAX is an acronym for Asynchronous JavaScript and XML. AJAX enables us
to send and receive data asynchronously without reloading the web page. AJAX is
used not only by many well known IT companies like Gmail, Google Maps, Youtube,
Facebook but by almost every company nowadays.
Example –
Google was one of the first major
companies to start using AJAX, and Google’s search suggestion tool was one of
the first ways they used it, and one of the first auto-complete tools made.
When typing into the Google search bar, it starts to use AJAX to get common
results from the database on each keystroke.
Q 2. What are the
advantages of using AJAX?
Ans 2. (A) Response time is faster so increases performance and speed.
(B)
Reduce the traffic travels between the client and the server.
(C)
Page not required to be reloaded
Q 3. How to use AJAX using
JQuery?
Ans 3. JQuery offers many methods that enable us to use AJAX in an effective
manner. You
can request text, HTML, XML, or JSON from a remote server using both HTTP Get
and HTTP Post and you can load the external data directly into the selected
HTML elements of your web page!
Q 4. How to use JQuery AJAX load method?
Ans 4. The load method loads data from a server and puts the returned data into
the selected element.
URL(required) – the URL where you want to load data from
data(optional) – It specifies a set of query string key/value pairs to send along with the request
callback(optional) – It is the name of the function to be executed after the load method is completed
Example – Suppose I have a text file(JQueryLoadTest.txt) on server having content as shown below –

Q 5. How to use JQuery AJAX get method?
Ans 5. The
get JQuery method requests data from the server with an HTTP GET
request.
SYNTAX – $.get(URL, callback)
URL(required) – the URL you want to requestcallback(optional) – It is the name of the function to be executed if the request is successful
EXAMPLE – Suppose you are using
ASP.NET MVC project and you have an action method “GetString” inside a
controller that returns a string “JQuery Tutorial” as shown below -
Now, if you want to get this string on button
click using AJAX then use,
$(“button”).click(function(){
$.get(ActionMethodURL,
function(data){
alert(data);
});
});
This would show an alert – “JQuery Tutorial”
Q 6. How to use JQuery AJAX post method?
Ans 6. The
post JQuery method requests data from the server with an HTTP POST
request.
SYNTAX – $.post(URL, data, callback)
data(optional) – It specifies some data to send along with the request
callback(optional) – It is the name of the function to be executed if the request is successful
EXAMPLE – Suppose you are using
ASP.NET MVC project and you have an action method “ChangeString” inside a
controller that accepts a string and then returns it after changing it as shown
below -
You can Post a string to this action method and
then get a changed string through JQuery POST method when user clicks a
button -
$(“button”).click(function(){
$.post(ActionMethodURL, ‘JQuery Tutorial’, function(data){
alert(data);
});
});
We are posting the string “JQuery Tutorial” but
we get the string – “Tech Geek” and it is alerted.
Q 7. How to use JQuery ajax
method?
Ans 7. The
ajax JQuery method is used when some desired action cannot be
obtained with other JQuery AJAX methods discussed before. For example, we want
to specify what should happen in case an Ajax call fails.
URL(required) – the URL you want to request
options – options is an object literal containing the configuration for the Ajax request.
EXAMPLE – You can now handle the successful posting of data as well as an error while doing so. With JQuery $.get() and $.post() methods you could not handle error scenario.
$(“button”).click(function(){
$.ajax(url:ActionMethodURL, method: “POST”, data: {Name:”Tech Geek”},
success:function(data){
alert(“data
posted successfully”);
},
error:function(JQxhr,
exception){
alert(“error occurred while posting data”);
});
});
No comments:
Post a Comment