JQuery Interview Questions Part - 5
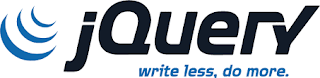
Q 1. What is DOM?
Ans 1. The
DOM defines a standard for accessing documents: “The W3C Document Object
Model(DOM) is a platform and language-neutral interface that allows programs
and scripts to dynamically access and update the content, structure, and style
of a document.”
Q 2. What is DOM
Manipulation?
Ans 2. DOM
Manipulation is the process of updating the content, structure, or style of a
document.
Q 3. How is DOM
Manipulation achieved in JQuery?
Ans 3. JQuery offers various DOM related methods that help manipulate HTML
elements and attributes. You can get, set, add, remove HTML elements and
attributes.
Q 4. What are the various
DOM Manipulation methods available in JQuery?
Ans 4. The
various DOM related methods available in JQuery are as follows –
·
$(selector).text()
·
$(selector).html()
·
$(selector).val()
·
$(selector).attr()
·
$(selector).append()
·
$(selector).prepend()
·
$(selector).after()
·
$(selector).before()
·
$(selector).remove()
·
$(selector).empty()
·
$(selector).addClass()
·
$(selector).removeClass()
·
$(selector).toggleClass()
·
$(selector).css()
Q 5. How is JQuery text
method used?
Ans 5. The
text JQuery method enables you to set or get the text content of the selected
HTML element.
Syntax –
$(selector).text()
Example –
$(“p”).text();
The above code gets the paragraph element text.
$(“p”).text(“This is a paragraph”);
The above code sets the paragraph element text to - "This is a paragraph".
Q 6. How is JQuery html
method used?
Ans 6. The
html JQuery method enables you to set or get the content of the selected HTML
element (including HTML mark-up).
Syntax –
$(selector).html()
Example –
$(“p”).html();
The above code gets the html content of the
paragraph element.
$(“p”).text(“This <b>is</b> a paragraph”);
The above code sets the html content of the
paragraph element to –“ This <b>is</b> a paragraph”.
Q 7. How is JQuery val
method used?
Ans 7. The
val JQuery method enables you to set or get the value of the selected form
fields.
Syntax –
$(selector).val()
Example –
$(“input”).val();
The above code gets the value of the input
element.
$(“input”).val(“test”);
The above code sets the value of the input
element to “test”.
Q 8. How is JQuery attr
method used?
Ans 8. The attr JQuery method is used to set or get the values of attributes of
selected HTML elements.
Syntax –
$(selector).attr(“Attribute_Name”)
Example –
$(“a”).attr(“href”);
The above code gets the value of “href” attribute
of the “a” element.
$(“a”).attr(“href”, “www.google.com”);
The above code sets the value of “href” attribute
of the “a” element to – “www.google.com”.
Q 9. How is JQuery append
method used?
Ans 9. The
append JQuery method inserts content at the end of the selected HTML element.
Syntax –
$(selector).append()
Example –
$(“p”).append(“<b>Append text</b>”);
The above code inserts content at the end of all "<p>" elements.
Q 10. How is JQuery prepend
method used?
Ans 10. The
prepend JQuery method inserts content at the beginning of the selected HTML
element.
Syntax –
$(selector).prepend()
Example –
$(“p”).prepend(“<b>Prepend text</b>”);
The above code inserts content at the beginning
of all "<p>" elements.
Q 11. How is JQuery after
method used?
Ans 11. The
after JQuery method inserts content after the selected HTML element.
Syntax –
$(selector).after()
Example –
$(“p”).after(“<b> Hello</b>”);
The above code inserts content after all "<p>" elements.
Q 12. How is JQuery before
method used?
Ans 12. The
after JQuery method inserts content before the selected HTML
element.
Syntax –
$(selector).before()
Example –
$(“p”).before(“<b> Hello</b>”);
The above code inserts content before all "<p>" elements.
Q 13. How is JQuery remove
method used?
Ans 13. The
remove JQuery method removes the selected HTML elements and its child elements.
Syntax –
$(selector).remove()
Example –
$(“p”).remove();
The above code removes all <p> elements and their child elements.
Q 14. How is JQuery empty
method used?
Ans 14. The
empty JQuery method removes the child elements from the selected HTML elements.
Syntax –
$(selector).empty()
Example –
$(“div”).empty();
The above code removes all the child elements from
the parent “<div>” elements.
Q 15. How is JQuery addClass
method used?
Ans 15. The
addClass JQuery method adds one or two css classes to the selected
HTML elements.
Syntax –
$(selector).addClass()
Example –
$(“p”).addClass(“FillColor”);
The above code adds a CSS class – “FillColor” to
all the “<p>” elements.
Q 16. How is JQuery
removeClass method used?
Ans 16. The
removeClass JQuery method removes one or more CSS classes
from the selected HTML elements.
Syntax –
$(selector).removeClass()
Example –
$(“p”).removeClass(“FillColor”);
The above code removes the CSS class –
“FillColor” from all the “<p>” elements.
Q 17. How is JQuery
toggleClass method used?
Ans 17. The
toggleClass JQuery method toggles between adding or removing
css classes from the selected HTML elements.
Syntax –
$(selector).toggleClass()
Example –
$(“p”).toggleClass(“FillColor”);
The above code toggles between adding and
removing the CSS class – “FillColor” to and from all the “<p>” elements.
Q 18. How is JQuery css
method used?
Ans 18. The
css JQuery method sets or gets the style for an HTML element.
Syntax –
$(selector).css()
Example –
$(“p.test”).css(“background-color”);
The above code gets the value of the css property
“background-color” for the “<p>” element that has id – “test”.
Example –
$(“p.test”).css(“background-color”, “red”);
The above code sets the value of the css property
“background-color” for the “<p>” element that has id – “test” to “red”.
No comments:
Post a Comment